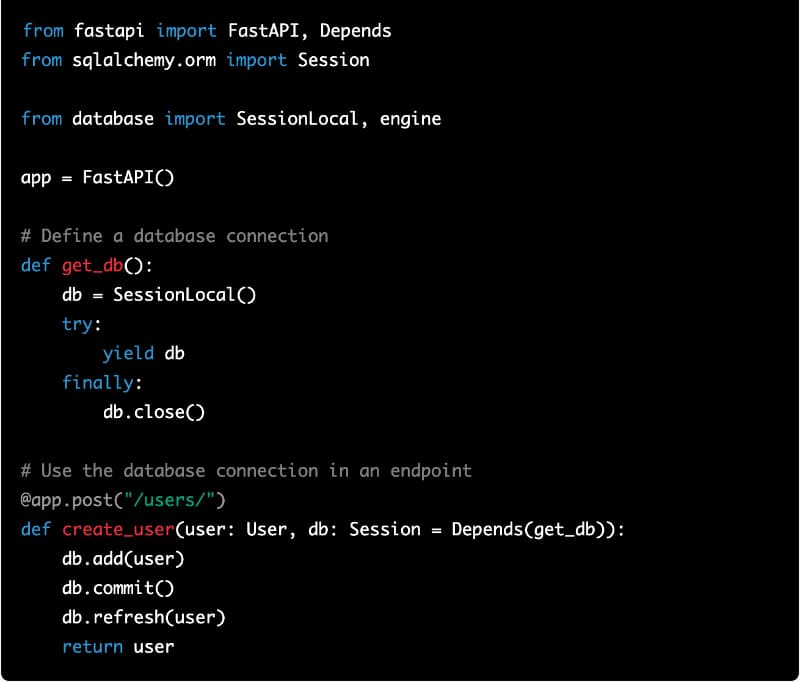
FastAPI is a modern, high-performance web framework for building APIs with Python 3.7+. It is based on the popular Python library called Starlette, and is built on top of the asyncio library, which allows it to handle a large number of concurrent connections efficiently. FastAPI is designed to be easy to learn and use, with a simple, intuitive API and a number of useful tools such as dependency injection and background tasks.
One of the standout features of FastAPI is its automatic documentation and validation. Using the Python type hints system, FastAPI is able to generate a complete documentation page for your API, complete with examples and validation rules. This makes it easy for developers to use and understand your API, as they can see exactly what is expected in each request and response. FastAPI also includes a built-in request validation system that ensures incoming requests meet the requirements specified in the type hints.
In addition to its documentation and validation capabilities, FastAPI is also designed to be fast and efficient. It is built on top of the Uvicorn and Hypercorn servers, which are production-ready and able to handle a large number of concurrent connections. This makes FastAPI well-suited for building high-performance APIs that can handle a large number of requests.
To get started with FastAPI, you will need to have Python 3.7+ installed on your machine. You can then install FastAPI using the Python package manager, pip.
Once FastAPI is installed, you can start building your first API. Here is a simple example of a FastAPI application:
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
This example creates a new FastAPI app and defines a single endpoint at the path "/"
. When a GET request is made to this endpoint, the read_root
function will be called and a simple JSON response will be returned.
To run this app, you can use the uvicorn
command:
uvicorn main:app –reload
This will start the app, and you can visit http://localhost:8000
in your web browser to see the response.
In addition to simple endpoints, you can also use FastAPI to build more complex APIs with multiple endpoints and dependencies. For example, you can use FastAPI’s dependency injection system to define a database connection and use it in your endpoints:
from fastapi import FastAPI, Depends
from sqlalchemy.orm import Session
from database import SessionLocal, engine
app = FastAPI()
# Define a database connection
def get_db():
db = SessionLocal()
try:
yield db
finally:
db.close()
# Use the database connection in an endpoint
@app.post("/users/")
def create_user(user: User, db: Session = Depends(get_db)):
db.add(user)
db.commit()
db.refresh(user)
return user
In this example, the get_db
function creates a new database connection and returns it to be used in the create_user
endpoint. This allows you to reuse the connection for multiple database operations without having to create a new connection each time.
FastAPI also includes support for background tasks, which allows you to run tasks in the background while the main application continues to handle requests. This is useful for tasks that take a long time to complete, or for tasks that need to be run periodically. To use background tasks in FastAPI, you can use the @background_task
decorator. Here is an example:
from fastapi import FastAPI, background_task
app = FastAPI()
@app.get("/long-task/")
@background_task
def long_task():
# Do some long-running work
for i in range(10):
time.sleep(1)
return {"result": "Task complete"}
In this example, the long_task
function will run in the background while the main application continues to handle requests. When the task is complete, the result will be returned to the client.
FastAPI is a powerful and flexible web framework that is well-suited for building APIs with Python. Its support for automatic documentation and validation, high-performance servers, and useful tools such as dependency injection and background tasks make it a popular choice for developers. If you’re looking to build a fast and efficient API with Python, FastAPI is definitely worth considering.